Python is a powerful and versatile programming language that offers many built-in features for developers. Mutable and immutable objects are two of the most important concepts in Python. Mutable objects can be changed after they are created, while immutable objects cannot. Understanding the difference between these two types of objects is essential for writing efficient and reliable code.
In this blog post, we will discuss the difference between mutable and immutable objects in Python. We will also provide examples of each type of object and discuss their uses. By the end of this blog post, you will have a solid understanding of the mutability concept and how to use it in your Python code.
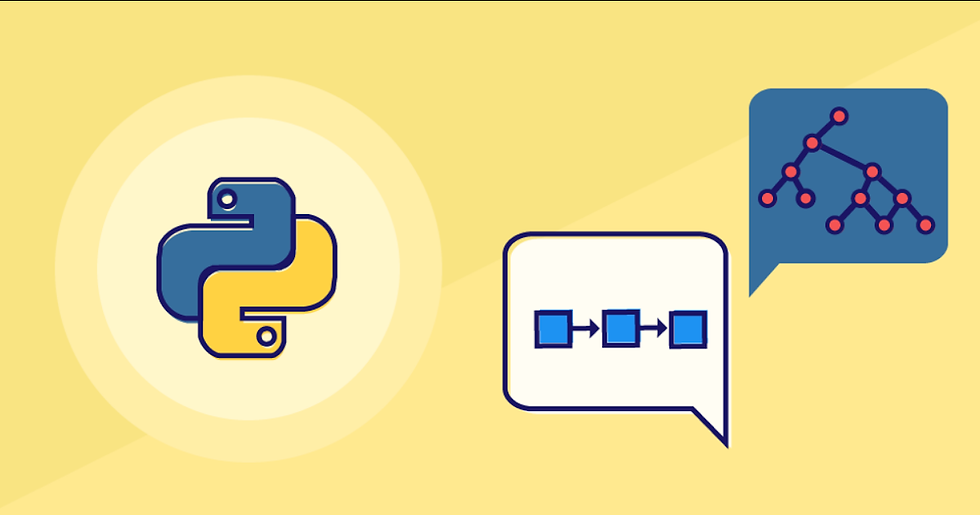
Mystery of Python Objects
We have all heard the famous statement "Everything is object in python". But a more logical statement would be "All Data is abstracted in Python using objects". Each object has an identity which is kind of an integer representation of its location in memory. Python has a command id() to get this integer.
>>> a = 123
>>> id(a)
4304100032
Python provides a number of datatypes few of which are mutable and others are immutable. Numbers, string, tuples are immutable while list, dicts are mutable. The hidden beauty of python is revealed when we explore mutable objects a little deeper. Lets say we have some value which is assigned to two variables.
>>> a = 1
>>> b = 1
If we process the above two statements we can say that two memory locations would be created for a and b where 1 will be stored. Lets check the integer representation of these memory locations.
>>> id(a)
4337357760
>>> id(b)
4337357760
Wait a minute. How can two memory locations have the same address ? Let me check with some other datatype. This time let me check with a list.
>>> l = [1, 2, 3]
>>> m = [1, 2, 3]
>>> id(l)
4336796544
>>> id(m)
4336232256
Hmmm something seems off here. So i received the same memory address for integer value but im receiving different memory address for lists. What is causing this change ? The answer lies in the definition of mutable and immutable objects. Integer being an immutable object cannot change its value hence it can be safely shared between multiple variables. So in order to save memory by storing the same value twice Python smartly shares this immutable value. But in case of mutable objects like lists and dicts since they are allowed to change their value hence they are not shared between variables.
Conclusion
Python being a highly abstracted language there are many important concepts which remain hidden from our sight. Knowing about such concepts help in better understanding about the language and write optimized and efficient code.
Comments